A simple example of interaction between WinForms and an expert written in MQL5.
Let’s create our test form in VisualStudio.
Follow all the steps according to the screenshots.
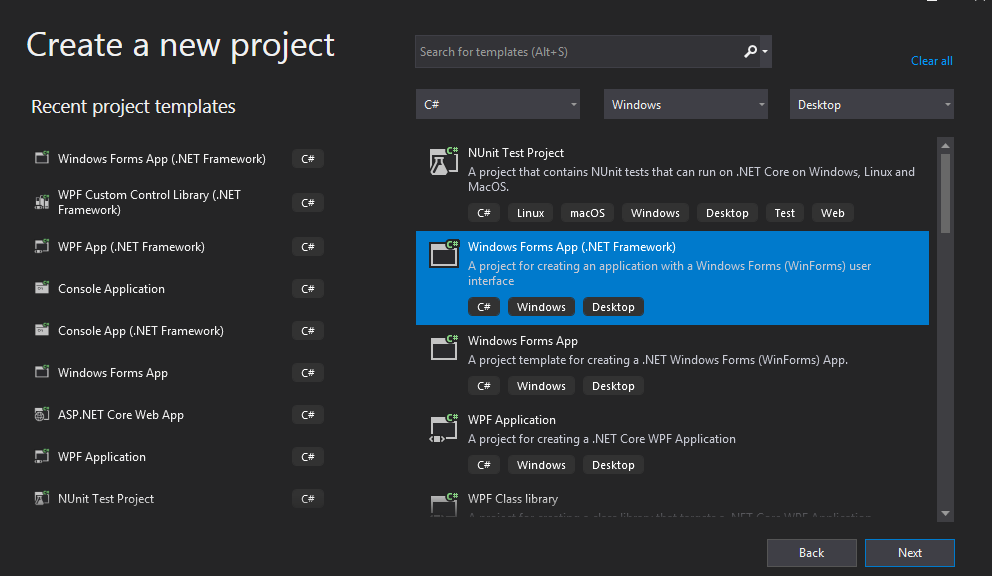
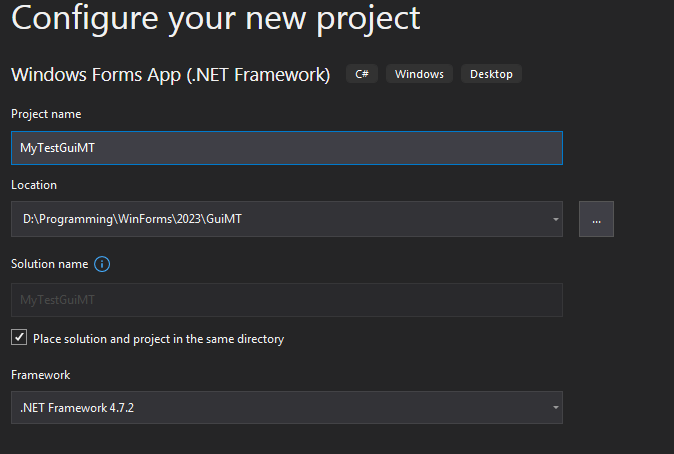
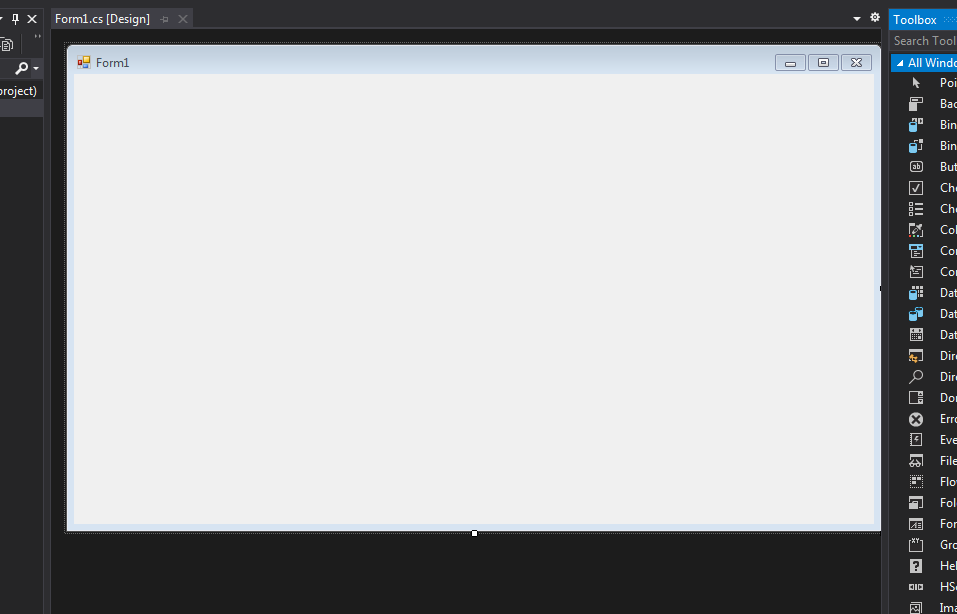
As a result, we will have this form. Let’s place several test buttons on it.
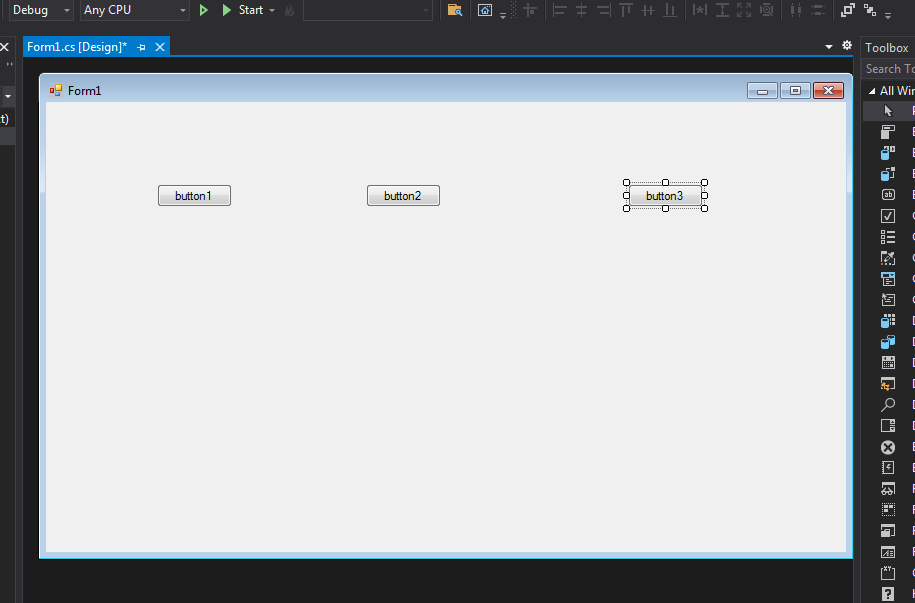
Let’s compile our form.
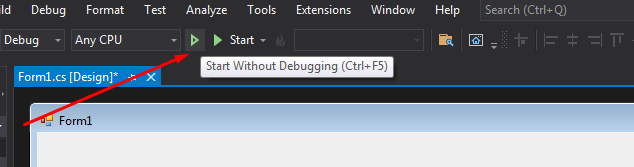
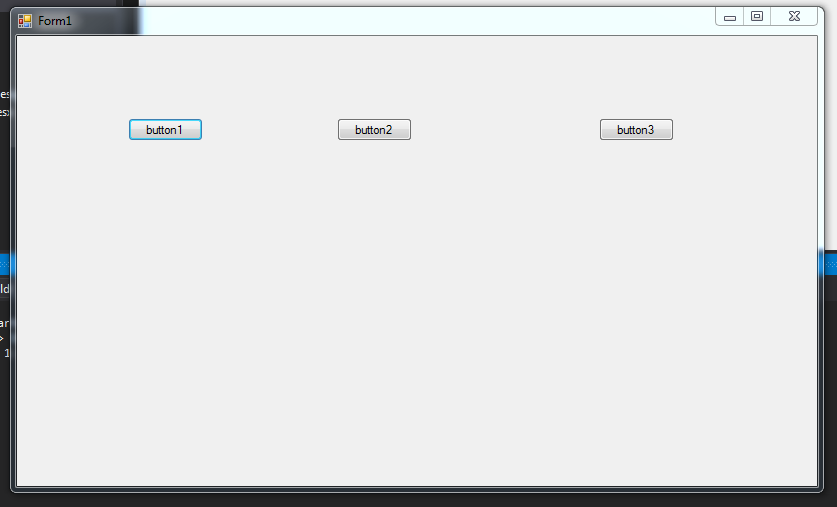
We need the absolute path to the executable form file.
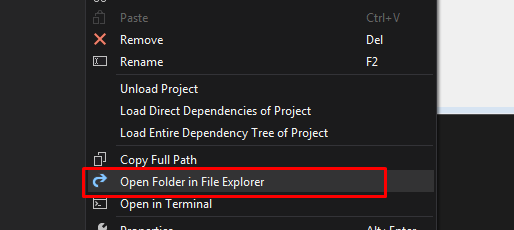
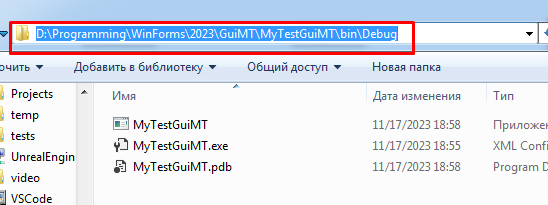
Copy this dll-file to the “\libraries” folder of your terminal. In my case this folder is located in the following path:

Now let’s go to MetaEditor5
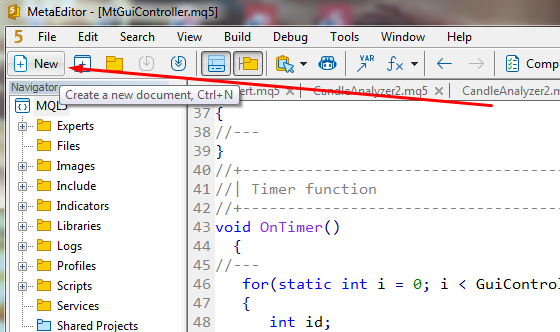
Create new expert.
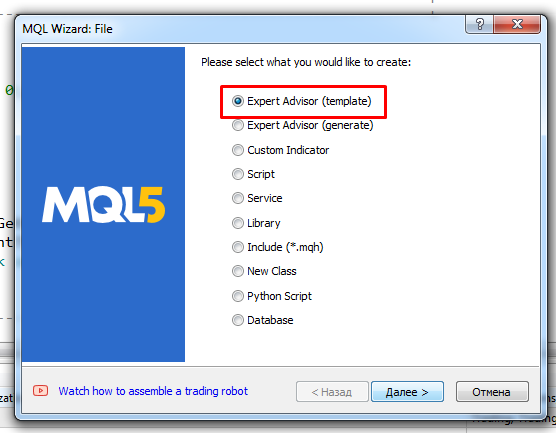
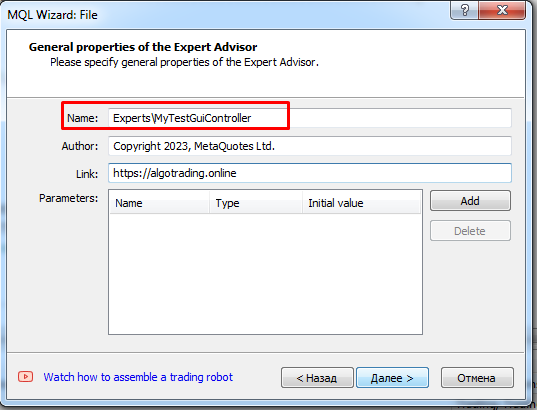
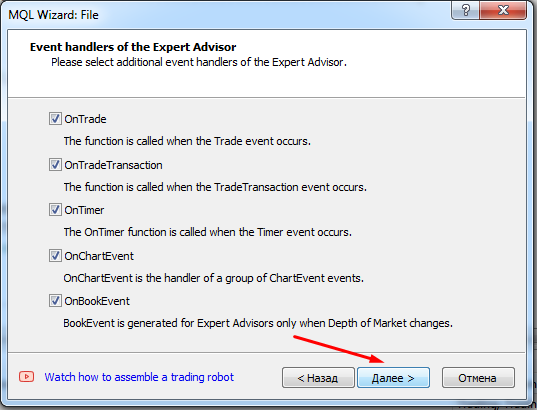
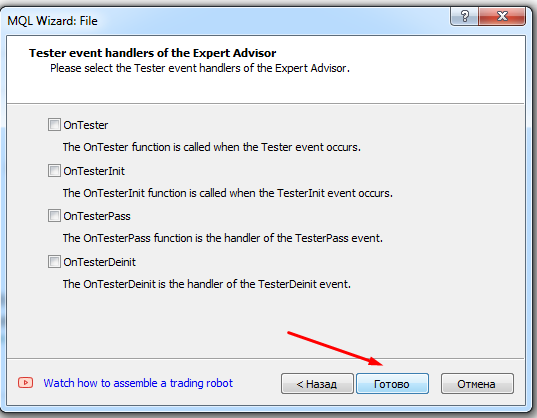
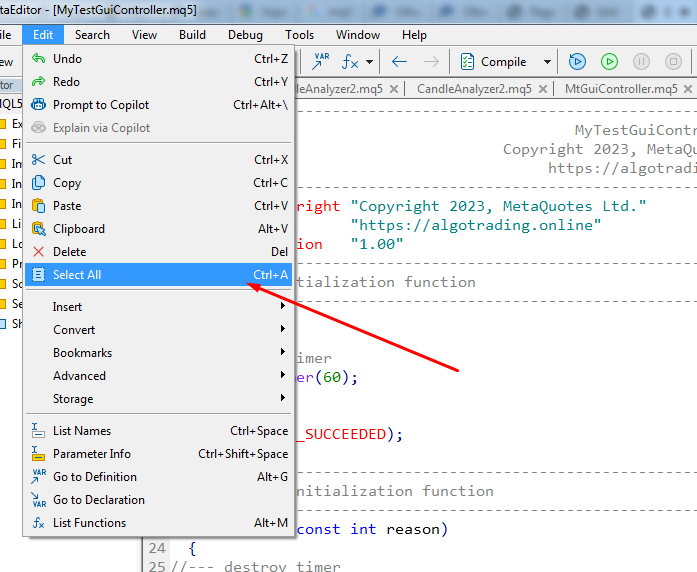
//+------------------------------------------------------------------+
//| GuiMtController.mq5 |
//| Copyright 2019, algotrading.online |
//| https://algotrading.online |
//+------------------------------------------------------------------+
#property copyright "Copyright 2018, MetaQuotes Software Corp."
#property link "https://algotrading.online"
#property version "1.00"
#import "MtGuiController.dll"
string assembly = "D:/Programming/WinForms/2023/GuiMT/MyTestGuiMT/bin/Debug/MyTestGuiMT.exe";
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//--- create timer
EventSetMillisecondTimer(200);
GuiController::ShowForm(assembly, "Form1");
//---
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
//--- destroy timer
GuiController::HideForm(assembly, "Form1");
EventKillTimer();
}
//+------------------------------------------------------------------+
//| Expert tick function |
//+------------------------------------------------------------------+
void OnTick()
{
//---
}
//+------------------------------------------------------------------+
//| Timer function |
//+------------------------------------------------------------------+
void OnTimer()
{
//---
for(static int i = 0; i < GuiController::EventsTotal(); i++)
{
int id;
string el_name;
long lparam;
double dparam;
string sparam;
GuiController::GetEvent(i, el_name, id, lparam, dparam, sparam);
if(id == GuiEventType::ClickOnElement)
printf("Click on element " + el_name);
}
}
//+------------------------------------------------------------------+
Copy this code and past in MetaEditor
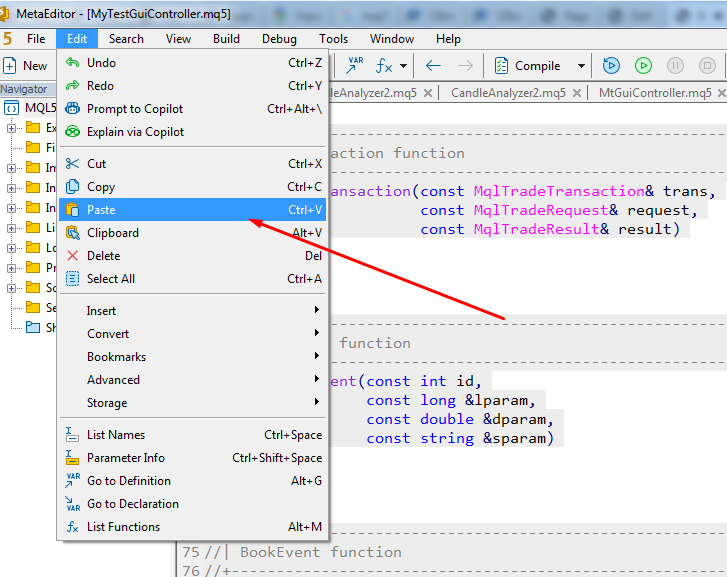
In this line you need to write the absolute path to the executable file of your form.

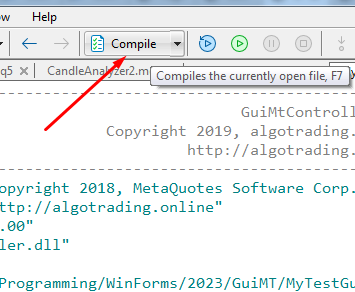
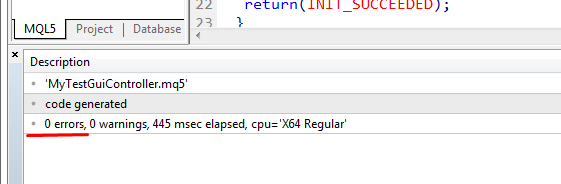
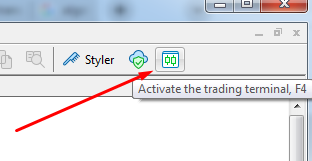
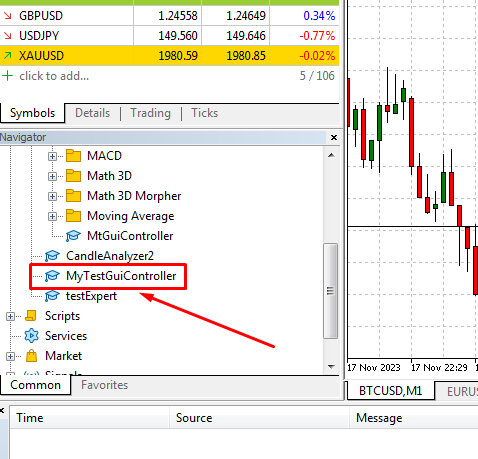
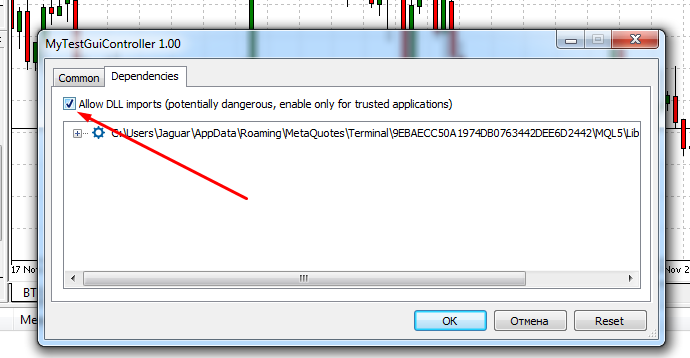
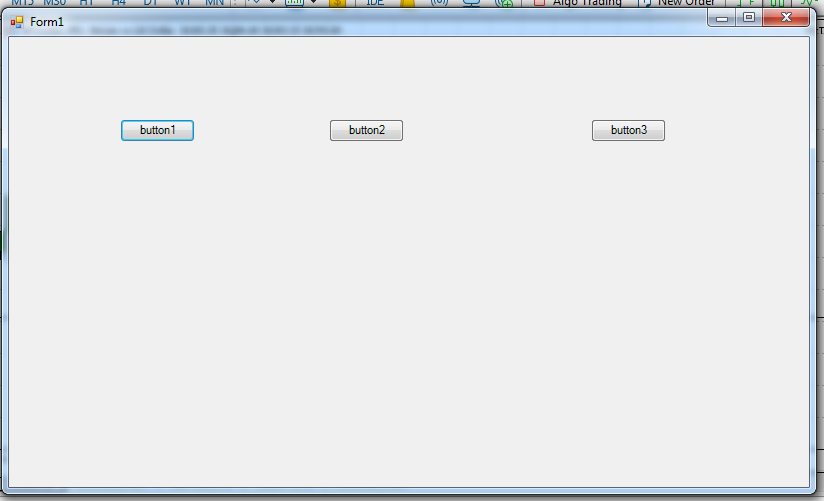
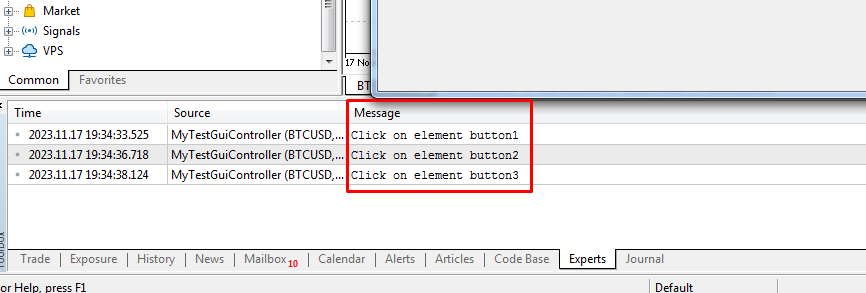
As you can see, our expert receives information about events that occurred in the WinForms form.
Now modify the code in the MQL5 program starting from line 54 as follows:
if(id == GuiEventType::ClickOnElement)
{
printf("Click on element " + el_name);
if(el_name=="button1") printf("Click on button1");
if(el_name=="button2") printf("Click on button2");
}
This code demonstrates that the Expert Advisor can perform certain actions based on events received from WinForms.
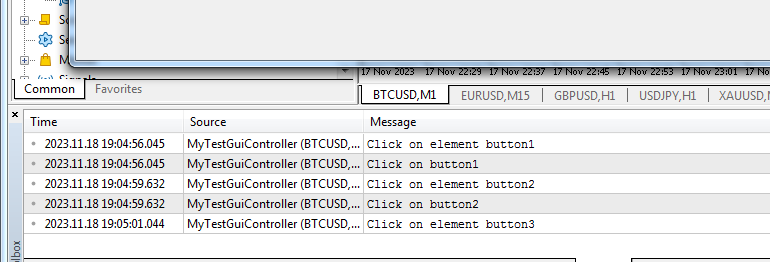
Now let’s specify a relative path instead of an absolute one. To do this, add the following code:
string assembly;
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//--- create timer
string mt_data_path = TerminalInfoString(TERMINAL_DATA_PATH);
string directory;
StringReplace(mt_data_path, "\\", "/");
directory = mt_data_path;
StringAdd(mt_data_path, "/MQL5/Libraries/GuiMT.exe");
assembly = mt_data_path;